Creating New Service Requests
Overview
This guide explains how to create New Service Requests for Licenses and Payer Enrollments.
Creating License New Service Requests
Preparation
Start by setting your Organization ID (reach out on Intercom if you've lost this UUID) and your API key. Then, gather your Provider's unique ID.
Save your IDs!
Provider IDsWe recommend storing your Providers' IDs when you invite them to Medallion (see Managing User Memberships and Importing / Adding Providers). However, if at any point you've lost track of them, you can use the GET Providers endpoint with a
search
to find them again, e.g. withrequests.get(providers_url, params={"search": "[[email protected]](mailto:[email protected])"}, headers=headers)
Service Request IDsAs with Provider IDs, we recommend storing the IDs of your Service Requests when you create them. However, if you've lost track of them, you can use either the GET Payer Enrollment Service Requests endpoint, the GET Credentialing Service Requests, or the GET Service Requests endpoint with the appropriate filters (e.g.
provider
orprovider_id
) to search for a given Service Request.
Through the Medallion API Platform UI
You can easily use the Medallion API Platform UI for creating License New Service Requests
Go to License Service Request endpoint. You can create new license requests for multiple states and multiple license types in a single request.
In order to create a new request you are required to fill
- Organization ID (
organization_id
) - Provider ID (
provider
) - Certificate Type (
certificate_type
)
Currently we allow making new requests for
License Type | Body Parameter |
---|---|
State Licenses | license_states |
DEA Registrations | dea_states |
CSR Registrations | csr_states |
State Registered Nurse Licenses | rn_states |
State Prescriptive Authority | prescriptive_authority_states |
State Autonomous Registration | autonomous_registration_states |
If you are using the endpoints through the Medallion API Platform UI, for each license type you want to request for, find the corresponding body parameter, click 'Add String' and select the state. It will look like the image below.
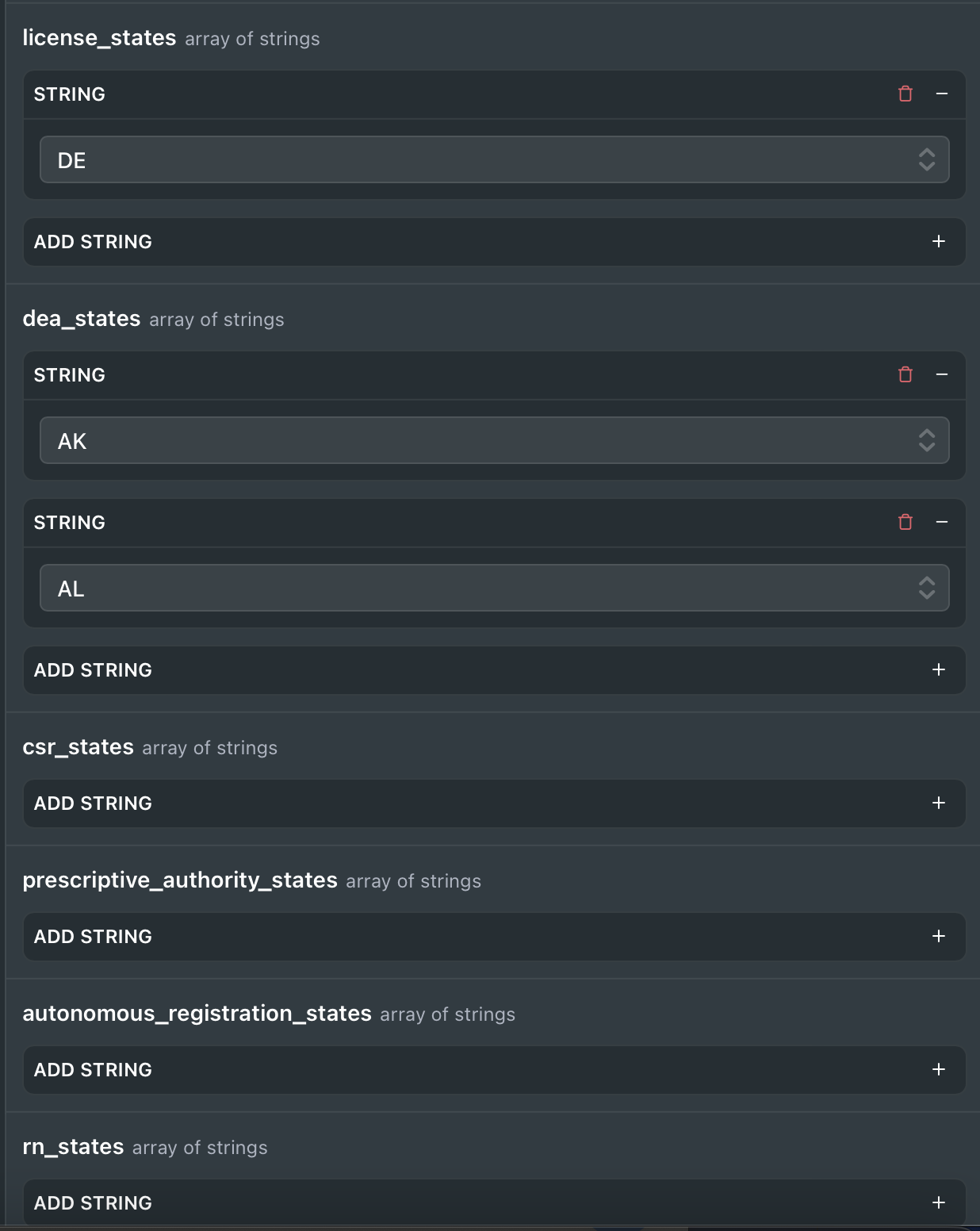
You can also specify whether the request will be owned and worked by Medallion or your organization. If you set
"is_medallion_owned": True
the request will be owned by Medallion
"is_medallion_owned": False
the request will be owned by your organization
Other optional body parameters are
-
duration_type
unless a different duration type is specified, request will be sent as `permanent`
-
created
-
request_via_imlc
-
request_supporting_nlc
Outside of the Medallion API Platform UI
If you are not using the Medallion API Platform UI, you need to specify the license type and add create a list of states
dea_states = ["AK", "AL"],
license_states = ["DE"]
Putting it all together
import requests
headers = {
"accept": "application/json",
"content-type": "application/json",
"x-api-key": "<YOUR_API_KEY>"
}
organization_id = "<YOUR_ORG_ID>"
provider_id = "<PROVIDER_ID>"
certificate_type = "<CERTIFICATE_TYPE>" #e.g. DO
#make a list of states you want for any of the available license types
dea_states = ["AK", "AL"],
license_states = ["DE"]
#optional parameters
request_via_imlc = True, #or False
request_supporting_nlc = True, #or False
created = "YYYY-MM-DD"
url = f"https://api.medallion.co/p/api/v1/organizations/{organization_id}/new-license-request/"
payload = {
"provider": provider_id
"certificate_type": certificate_type,
#your license types you create requests for
"dea_states": dea_states,
"csr_states": license_states,
#if you set any optional parameters include them here
"duration_type": "training", #set to "permanent" by default unless specified otherwise
"request_via_imlc": request_via_imlc,
"request_supporting_nlc": request_supporting_nlc,
"created": created
}
response = requests.post(url, json=payload, headers=headers)
response_json = response.json()
print(response_json)
Creating Payer Enrollment New Service Requests
You can create Payer Enrollment New Service Requests for a Group or a Provider.
You can easily use the Medallion API Platform UI for creating Payer Enrollment New Service Requests by going to POST a Payer Enrollment Service Request endpoint.
Preparation
- Gather your Payer's State and Name and also if you know your Payer's unique UUID. Set these parameters
state = "<YOUR_PAYER_STATE>"
payer_name = "<YOUR_PAYER_NAME>"
payer_id= "<YOUR_PAYER_ID>" #set if you know
is_medallion_owned = True #True if the request will be owned by Medallion, False if owned by your org
- You might want to add Lines of Business to your request. If you do not know IDs for lines of business, you can use the GET Lines Of Business endpoint and get the IDs corresponding to the business lines you want. If you are using the Medallion API Platform UI, you can click on ADD OBJECT under
lines_of_business
to add the each Line of Business ID you want. If you are not using the Medallion API Platform UI
lines_of_business = [{ "id": "<LINE_OF_BUSINESS_ID>" }, { "id": "<LINE_OF_BUSINESS_ID>" }]
- Similarly, you may want to add Practices to your request. If you are using the Medallion API Platform UI, you can click on ADD OBJECT under
practices
to add the each Line of Business ID you want. You need the ID of the Practice and other Practice parameters suchname
and address related parameters are optional. If you are not using the Medallion API Platform UI
practices = [
{
"id": "<PRACTICE_ID>",
"name": "<PRACTICE_NAME>",
"country": "<PRACTICE_COUNTRY>",
},
{
"id": "<PRACTICE_ID>",
}
],
New Request for a Group
Gather your Group Profile ID. If you did not saved your Group Profile ID, you can use the GET Group Profiles endpoint with a search
or name
to find it. Then set group_profile
with this ID.
If you are using the Medallion API Platform UI, please select NewGroupPayerEnrollmentServiceRequest body parameter.
If you are requesting outside of the the Medallion API Platform UI
import requests
headers = {
"accept": "application/json",
"content-type": "application/json",
"x-api-key": "<YOUR_API_KEY>"
}
group_profile="<GROUP_PROFILE_ID>"
state = "<YOUR_PAYER_STATE>"
payer_name = "<YOUR_PAYER_NAME>"
payer_id= "<YOUR_PAYER_ID>" #set if you know
is_medallion_owned = True #True if the request will be owned by Medallion, False if owned by your org
resourcetype= "NewGroupPayerEnrollmentServiceRequest"
#lines_of_business and practices are optional
lines_of_business = [{ "id": "<LINE_OF_BUSINESS_ID>" }, { "id": "<LINE_OF_BUSINESS_ID>" }]
practices = [
{
"id": "<PRACTICE_ID>",
"name": "<PRACTICE_NAME>",
"country": "<PRACTICE_COUNTRY>",
},
{
"id": "<PRACTICE_ID>",
}
]
url = "https://app.medallion.co/p/api/v1/service-requests/payer-enrollments/"
payload = {
"group_profile":group_profile,
"state":state,
"payer_name":payer_name,
"payer_id":payer_id,
"is_medallion_owned": is_medallion_owned,
"resourcetype": resourcetype,
"lines_of_business":lines_of_business,
"practices":practices
}
response = requests.post(url, json=payload, headers=headers)
response_json = response.json()
print(response_json)
New Request for a Provider
Gather your your Provider's ID. If you did not saved your Provider's ID, you can use the GET Providers endpoint with a search
to find them again, e.g. with requests.get(providers_url, params={"search": "[email protected]"}, headers=headers)
. Then set provider
with this ID.
If you are using the Medallion API Platform UI, please select NewProviderPayerEnrollmentServiceRequest body parameter.
import requests
headers = {
"accept": "application/json",
"content-type": "application/json",
"x-api-key": "<YOUR_API_KEY>"
}
provider = "<PROVIDER_ID>"
state = "<YOUR_PAYER_STATE>"
payer_name = "<YOUR_PAYER_NAME>"
payer_id= "<YOUR_PAYER_ID>" #set if you know
is_medallion_owned = True #True if the request will be owned by Medallion, False if owned by your org
resourcetype= "NewProviderPayerEnrollmentServiceRequest"
#lines_of_business and practices are optional
lines_of_business = [{ "id": "<LINE_OF_BUSINESS_ID>" }, { "id": "<LINE_OF_BUSINESS_ID>" }]
practices = [
{
"id": "<PRACTICE_ID>",
"name": "<PRACTICE_NAME>",
"country": "<PRACTICE_COUNTRY>",
},
{
"id": "<PRACTICE_ID>",
}
]
url = "https://app.medallion.co/p/api/v1/service-requests/payer-enrollments/"
payload = {
"provider":provider,
"state":state,
"payer_name":payer_name,
"payer_id":payer_id,
"is_medallion_owned": is_medallion_owned,
"resourcetype": resourcetype,
"lines_of_business":lines_of_business,
"practices":practices
}
response = requests.post(url, json=payload, headers=headers)
response_json = response.json()
print(response_json)
Updated about 1 year ago